Originally published on bendyworks.com.
With users now being able to sign in with Google, I want to have them confirm their name and agree to a terms of service. To lay the groundwork I'm going to add a placeholder RegisterPage
and navigation to it.
This will be my second page, of what I expect to be many, so I went ahead and created a pages
directory. The existing MyHomePage
class was renamed to HomePage
and relocated to the pages
directory. That's a refactor and ideally should be in its own pull request, but it's of minimal impact so I'm lumping in with today's other changes.
The new RegisterPage
is simple and currently just displays some text. On the first implementation I did find that the AppBar
title was no not centered.
This is because I was placing the text in a Center
widget. Center
was centering the text in the space available to it but the back navigation icon was not being accounted for.
AppBar(
title: Center(
child: Text('Register'),
),
elevation: 0.0,
)
~~~{% endraw %}
A quick switch to using {% raw %}`centerTitle`{% endraw %} fixes it.{% raw %}
~~~dart
AppBar(
title: Text('Register'),
centerTitle: true,
elevation: 0.0,
)
~~~{% endraw %}
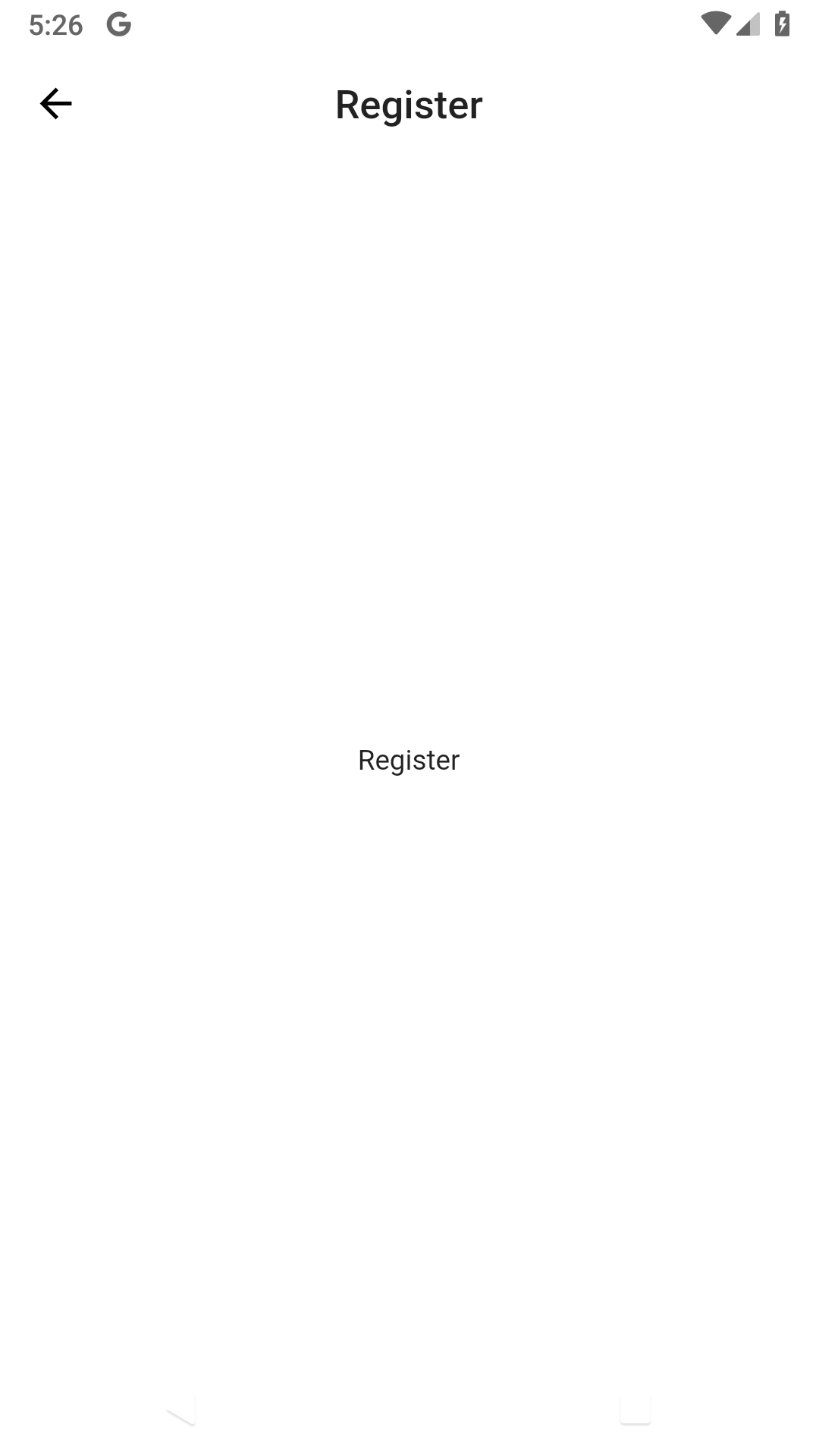
But how do users get to this new registration page? It has to happen after a user is authenticated (how else will I know if they are registered?) but before they perform any other authenticated actions. That only leaves {% raw %}`_handleSignIn`{% endraw %} in {% raw %}`SignInFab`{% endraw %}.{% raw %}
~~~dart
void _handleSignIn(BuildContext context) {
auth.signInWithGoogle().then((FirebaseUser user) {
if (_existingUser()) {
_showSnackBar(context, 'Welcome ${user.displayName}');
} else {
_navigateToRegistration(context);
}
});
}
~~~{% endraw %}
For now I've set {% raw %}`_existingUser` to return a hardcoded boolean. If they are an existing user they should just see the `SnackBar`, otherwise send them to the `RegisterPage`.
~~~dart
void _navigateToRegistration(BuildContext context) {
Navigator.pushNamed(context, RegisterPage.routeName);
}
~~~
`_navigateToRegistration` uses the [`Navigator` widget](https://docs.flutter.io/flutter/widgets/Navigator-class.html) to push a new route onto the stack by name. In this case I'm defining the {% raw %}`routeName`{% endraw %} in {% raw %}`RegisterPage`{% endraw %} itself so if I want to change it later, I only have to change it in one place.
In {% raw %}`MyApp`{% endraw %} I will now register the route with {% raw %}`MaterialApp`{% endraw %}, also using the {% raw %}`routeName`{% endraw %} from {% raw %}`RegisterPage`{% endraw %}.{% raw %}
~~~dart
MaterialApp(
home: const HomePage(title: 'Birb'),
routes: <String, WidgetBuilder>{
RegisterPage.routeName: (BuildContext context) => const RegisterPage(),
},
);
~~~{% endraw %}
## Code changes
- [#53 Add RegisterPage and route](https://github.com/abraham/birb/pull/53)
Top comments (0)