Liquid syntax error: Tag '{% raw %}' was not properly terminated with regexp: /\%\}/
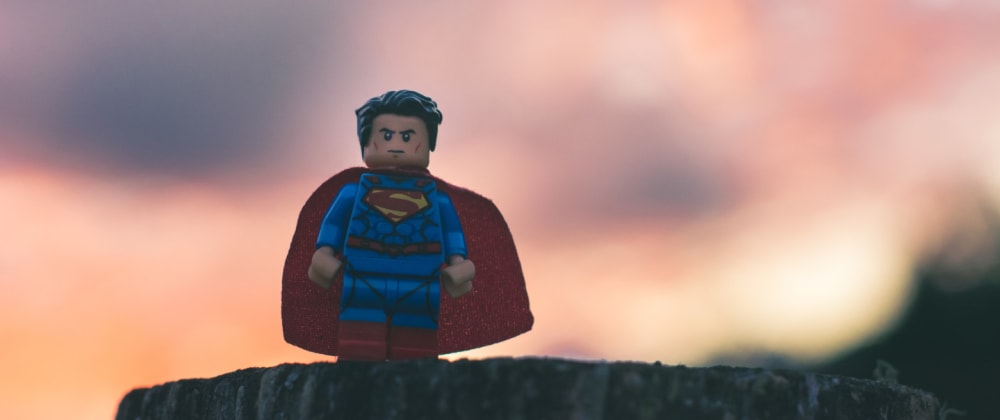
For further actions, you may consider blocking this person and/or reporting abuse
Posted on • Originally published at bendyworks.com
Liquid syntax error: Tag '{% raw %}' was not properly terminated with regexp: /\%\}/
For further actions, you may consider blocking this person and/or reporting abuse
Ciprian Popescu -
FullStackJava -
ByteHide -
AMatisse -
Top comments (0)