Liquid syntax error: Tag '{% raw %}' was not properly terminated with regexp: /\%\}/
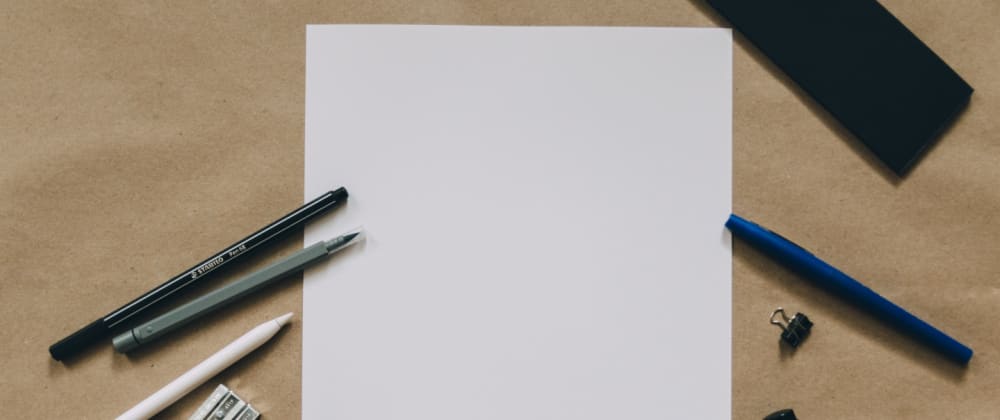
For further actions, you may consider blocking this person and/or reporting abuse
Liquid syntax error: Tag '{% raw %}' was not properly terminated with regexp: /\%\}/
For further actions, you may consider blocking this person and/or reporting abuse
Akshat Sharma -
Felipe Ishihara -
Rolf Streefkerk -
RaAj Aryan -
Top comments (0)